Ingress, Gateway, Load Balancer, ClusterIP, and NodePort... What the Heck Are They? A Simplified Guide to Kubernetes Services!
TL;DR
Kubernetes networking can be as confusing as... well, Kubernetes itself. Are you tired of getting distracted from your coding spree by kubernetes mess-ups? Here we offer a simple guide to Kubernetes Services, focusing on the key players: Ingress, Gateway, Load Balancer, ClusterIP, and NodePort. We'll unravel their purposes, strengths, and when to deploy each one.
Demystifying Kubernetes Services
The typical scenario: you've containerized your application using Docker, breaking it down into microservices spread across a Kubernetes cluster. But how do these microservices communicate? How does external traffic reach them?
Enter Kubernetes Services, the unsung heroes of the Kubernetes networking world. They act as internal load balancers and provide a stable endpoint to access your pods (those containers running your app). Think of them as the friendly traffic directors ensuring seamless communication between your application components.
Kubernetes offers several types of Services, each catering to specific needs:
ClusterIP: Your Application's Private Lounge
The most basic type is the ClusterIP service. It provides a single, stable IP address within your cluster, accessible only by other applications running inside the same cluster. It's like having a private party in your house where only those with the invitation (other pods in the cluster) can join.
Use ClusterIP when:
- You need internal communication between pods within your cluster.
- You don't need external access to the service.
Here's a simple example:
apiVersion: v1
kind: Service
metadata:
name: my-internal-app
spec:
type: ClusterIP
selector:
app: my-app
ports:
- port: 80
targetPort: 8080
In this example:
type: ClusterIP
explicitly defines the service type as ClusterIP.selector: app: my-app
tells the service to select pods with the labelapp: my-app
.ports:
define the port mappings between the service (port 80) and the pods (targetPort 8080).
NodePort: Punching a Hole Through the Firewall
Next up is NodePort, which exposes your service on a static port on every node in your cluster. It's like opening a designated door at your house party and letting anyone who knows the door number (the NodePort) in, whether they're on the guest list or not.
NodePort is useful for:
- Exposing your application for external access during development or debugging.
- Exposing a service on a specific port on all nodes, regardless of the underlying pod's IP address.
Here's how you'd define a NodePort service:
apiVersion: v1
kind: Service
metadata:
name: my-nodeport-service
spec:
type: NodePort
selector:
app: my-app
ports:
- port: 80
targetPort: 8080
nodePort: 30080
The key difference here is nodePort: 30080
. This line exposes the service on port 30080 on every node in your cluster.
LoadBalancer: The External Traffic Controller
While NodePort opens a door, LoadBalancer rolls out the red carpet, providing a dedicated external IP address that routes traffic to your service. It's like hiring a professional bouncer (often provided by your cloud provider) who expertly manages the guest list and ensures smooth entry to your party.
LoadBalancer services are ideal for:
- Exposing your application to the outside world in a production environment.
- Distributing traffic across multiple pods for high availability and scalability.
Here's an example:
apiVersion: v1
kind: Service
metadata:
name: my-loadbalancer-service
spec:
type: LoadBalancer
selector:
app: my-app
ports:
- port: 80
targetPort: 8080
When you create a LoadBalancer service, Kubernetes (with the help of your cloud provider) provisions a load balancer and assigns it an external IP address, making your application publicly accessible.
Ingress: The Intelligent Traffic Router
Think of LoadBalancers as providing a single entry point to your application. But what if you want more fine-grained control over incoming traffic, like routing requests to different services based on the requested URL?
Enter Ingress, the sophisticated traffic controller that acts as a reverse proxy, directing traffic to different services based on rules you define. Imagine Ingress as the party planner who greets guests at the door and guides them to different rooms (services) based on their interests (request URL).
Ingress shines when:
- You need to route traffic based on HTTP/HTTPS attributes like hostnames, paths, or headers.
- You want to consolidate multiple services under a single entry point (like a single domain name).
Here's a basic Ingress configuration:
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: my-ingress
spec:
rules:
- host: myapp.example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: my-service-1
port:
number: 80
- path: /admin
pathType: Prefix
backend:
service:
name: my-service-2
port:
number: 8080
This configuration routes requests to myapp.example.com/
to my-service-1
and requests to myapp.example.com/admin
to my-service-2
.
Gateway API: The Future of Kubernetes Networking
While Ingress has been the go-to for HTTP traffic routing, it has its limitations. Enter the Gateway API, a more powerful, expressive, and extensible way to manage Kubernetes networking. Think of Gateway API as the evolution of the party planner, now equipped with advanced skills and tools to handle even the most complex events.
Here's a simplified example:
apiVersion: gateway.networking.k8s.io/v1alpha2
kind: Gateway
metadata:
name: my-gateway
spec:
gatewayClassName: istio
listeners:
- protocol: HTTP
port: 80
---
apiVersion: gateway.networking.k8s.io/v1alpha2
kind: HTTPRoute
metadata:
name: my-route
spec:
parentRefs:
- name: my-gateway
hostnames:
- "myapp.example.com"
rules:
- matches:
- path:
type: PathPrefix
value: /
backendRefs:
- name: my-service-1
port: 80
The Gateway API provides a more structured and flexible way to define gateways, listeners, and routes, making it easier to manage complex networking scenarios.
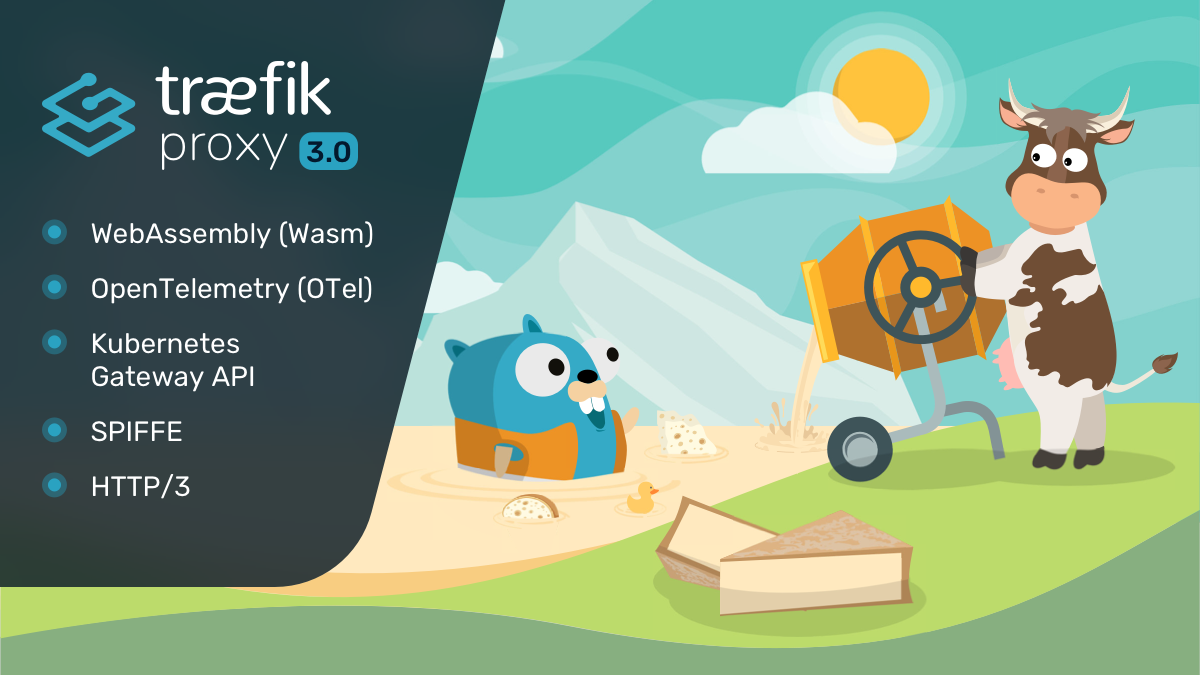
Choosing the Right Service Type
- Internal communication: ClusterIP is your go-to.
- External access during development: NodePort offers a simple solution.
- Production-ready external access with load balancing: LoadBalancer is your best bet.
- Traffic routing based on HTTP/HTTPS attributes: Ingress is the answer.
- Advanced traffic management and extensibility: Embrace the power of Gateway API.
Remember, understanding Kubernetes Services is key to unlocking the full potential of your containerized applications. Choose wisely, and your microservices will be chatting like old friends in no time!
Going Beyond the Basics: Service Meshes
For advanced networking and traffic management, consider exploring Service Meshes like Istio, Linkerd, Traefik Mesh, Kong or Kuma. These tools provide additional features like traffic encryption, observability, and fine-grained traffic control, taking your Kubernetes networking game to the next level.
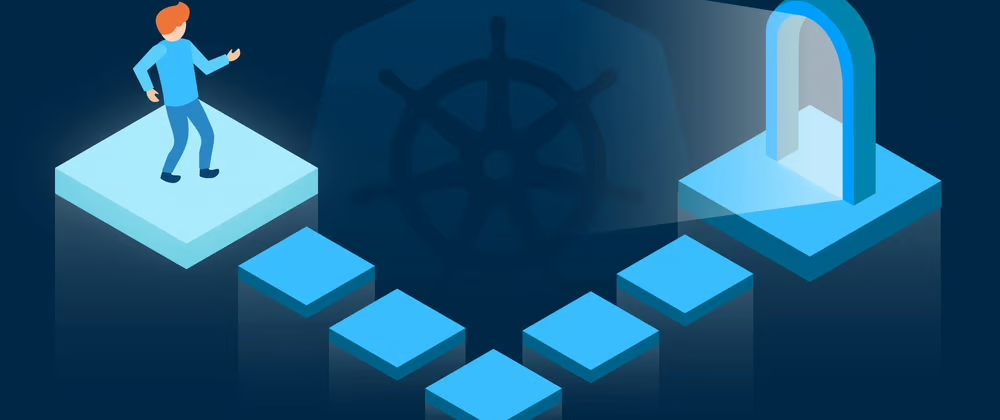
- Register with Email
- Login with LinkedIn
- Login with GitHub